Glossary
Async function
A function that returns a Promise. The await
keyword is only usable in an async function.
const wait = (ms) => new Promise((res) => setTimeout(res, ms));
const fn = async () => {
await wait(100);
return "result";
}
fn(); // Promise
Async generator function
A generator function that can use await
. When it encounters a yield
or a yield*
the execution stops until a subsequent element is requested.
const wait = (ms) => new Promise((res) => setTimeout(res, ms));
const gen = async function*() {
console.log("start");
await wait(100);
yield 1;
await wait(100);
console.log("next");
yield 2;
await wait(100);
console.log("end");
};
const it = gen();
console.log(await it.next());
console.log(await it.next());
console.log(await it.next());
// start
// { value: 1, done: false }
// next
// { value: 2, done: false }
// end
// { value: undefined, done: true }
See Generator function.
await keyword
Usable in async functions, it stops the execution until the argument Promise becomes fulfilled. It then returns the value or throws an exception. See Async function.
Callback
A function passed as an argument that is called with the return value. It allows asynchronous results, as the callback can be invoked later.
For example, the setTimeout
function needs a callback that it calls when the time is up:
setTimeout(() => {
// 1 second later
}, 1000);
See Continuation-passing style.
Collection processing
A series of functions that work on a collection of elements (usually, an array), such as transforming (map
), filtering (filter
), and reducing to a value (reduce
).
Continuation-passing style
A programming construct that uses callback functions (continuations) for function results instead of returning them directly. This allows asynchronous results as these callbacks can be invoked later.
// direct style
const directFn = () => {
return "result";
};
// CPS
const cpsFn = (callback) => {
callback("result");
};
See Callback.
Error propagation
When there is an error, it moves up in context until there is a piece of code that can handle it.
For example, an unhandled exception gets thrown to the caller:
const fn = () => {
// the error is thrown here
throw new Error("error");
}
try {
fn();
}catch(e) {
// and handled here
}
With Promises, errors propagate to the next handlers:
getUser() // returns a Promise
.then(() => {
// the error is thrown here
throw new Error("error");
})
.catch((e) => {
// and handled here
});
Error-first callbacks
A callback style where the first argument is an error object. The receiving end needs to check if this value is non-null (or non-undefined).
const fn = (callback) => {
try {
const result = "result";
// error is null
callback(null, result);
}catch(e) {
// signal error
callback(e);
}
}
fn((err, result) => {
if (err) {
// handle error
}else {
// handle result
}
});
See Node-style callbacks.
Fulfilled Promise
A state of a Promise that is either resolved or rejected. See Promise states.
Generator function
A function that returns an iterable structure. It can be frozen with a yield
statement and only resumes if more elements are requested.
const gen = function*() {
console.log("start");
yield 1;
console.log("next");
yield 2;
console.log("end");
}
const it = gen();
console.log(it.next());
console.log(it.next());
console.log(it.next());
// start
// { value: 1, done: false }
// next
// { value: 2, done: false }
// end
// { value: undefined, done: true }
Node-style callbacks
A callback style where:
- The callback function is the last argument
- It is called with an error object first, then a result (
(error, result)
) - It returns only one result
const getUser = (id, cb) => {
const user = /* get user */;
if (user) {
// success
cb(null, user);
}else {
// error
cb(new Error("Failed to get user"));
}
};
Pagination
When a method (usually an API) does not return all results for a request and it requires multiple calls to get all items.
Pending Promise
A Promise state where the asynchronous result is not yet available. See Promise states.
postMessage
An API to communicate cross-context, such as with IFrames and Web Workers. It is an event-based communication where one end listens for message
events and the other uses the postMessage
function to send messages.
Promise
A value that holds an asynchronous result. You can use the then
callback to handle when the Promise becomes fulfilled, or use await
in an async function.
Promise chain
A series of then()
callbacks, each getting the previous state of the Promise and returns the next one.
getUser() // returns a Promise
.then(async function getPermissionsForUser(user) {
const permissions = await // ...;
return permissions;
})
.then(async function checkPermission(permissions) {
const allowed = await // ...;
return allowed;
})
.then((allowed) => {
// handle allowed or not
});
Promise states
A Promise can be in one of multiple states:
- Pending: The value is not yet available
- Resolved: The value is available
- Rejected: There was an error
When the Promise is either resolved or rejected then it's fulfilled or settled.
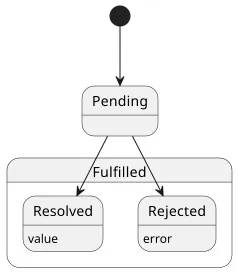
Promise.all
A utility function that gets multiple Promises and resolves when all of them are resolved and returns an array with the results. It rejects if any of the input Promises rejects.
console.log(
await Promise.all([
Promise.resolve("a"),
Promise.resolve("b"),
])
);
// ["a", "b"]
Promise.allSettled
A utility function that gets multiple Promises and resolves when all of them are settled. It resolves even if some input Promises reject.
console.log(
await Promise.allSettled([
Promise.resolve("a"),
Promise.reject("b"),
])
);
// [
// { status: 'fulfilled', value: 'a' },
// { status: 'rejected', reason: 'b' }
// ]
Promise.catch
A utility function that attaches a rejection handler to a Promise. It's equivalent to then(undefined, handler)
.
Promise.reject(new Error("error"))
.catch((e) => {
console.log(e.message); // error
});
Promise.finally
A utility function that is called when the Promise settles, either if it's resolved or rejected. It brings the try..finally
construct to Promises.
getUser() // returns a Promise
.finally(() => {
// called both even if the Promise is rejected
console.log("finished");
});
// does not change the result value
Promise.race
A utility function that gets multiple Promises and resolves (or rejects) when the first one settles.
const wait = (ms) => new Promise((res) => setTimeout(res, ms));
const fn = async (ms, result) => {
await wait(ms);
return result;
}
console.log(
await Promise.race([
fn(100, "a"),
fn(50, "b"),
])
);
// b
Promise.reject
A utility function that rejects with the value provided.
Promise.reject(new Error("error"))
.catch((v) => {
console.log(v.message); // error
})
Promise.resolve
A utility function that returns a Promise that is resolved with the value provided. Useful to start a Promise chain.
Promise.resolve("value")
.then((v) => {
console.log(v); // value
})
Promise.then
A function of a Promise object that attaches a function that will be called when the Promise is settled. See Promise chain.
Promisification
The process of converting callbacks to Promises.
Rejected Promise
A Promise state that indicates an error. See Promise states.
Resolved Promise
A Promise state where the asynchronous value is available. See Promise states.
Settled Promise
An alternative name for the fulfilled state. See Promise states.
Synchronous function
A function that returns a value when it's run. See Continuation-passing style.